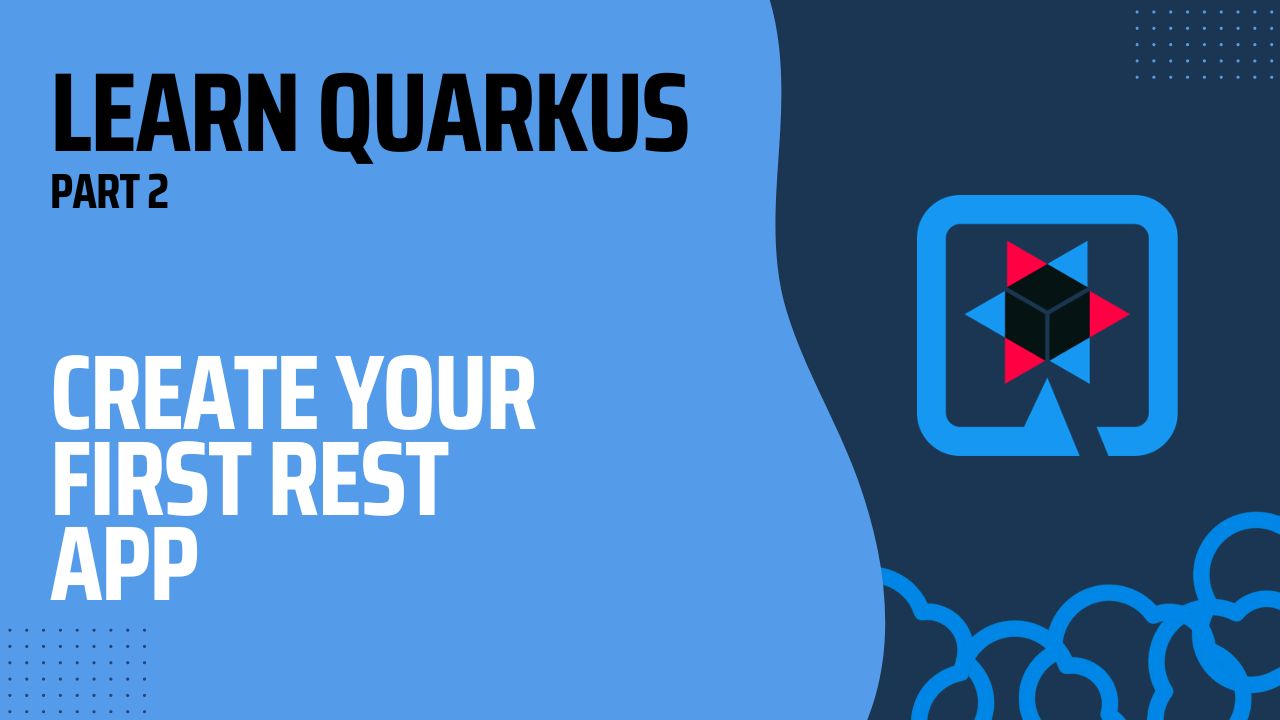
Building Your First Quarkus Application - Learn Quarkus Part 2
Introduction
After setting up your development environment for Quarkus in Part 1, it’s time to dive into creating your first application. This tutorial will guide you through the process of building a simple RESTful application using Quarkus, showcasing its ease of use and developer-friendly features.
Creating the Project
You can create a new Quarkus project either using the Quarkus CLI or through the Maven/Gradle plugin
- Generate a New Project:
- Open your terminal or command prompt
- To use Quarkus CLI:
- Install the CLI following this guide
- Run the command:
quarkus create app com.codevup:quarkus-intro-demo
- To use Maven plugin:
- Run the following command
mvn io.quarkus:quarkus-maven-plugin:2.11.3.Final:create \ -DprojectGroupId=com.codevup \ -DprojectArtifactId=quarkus-intro-demo \ -DclassName="com.codevup.quarkus.demo.GreetingResource" \ -Dextensions="resteasy" \ -Dpath="/hello"
- Run the following command
- This command creates a new Quarkus project in the my-quarkus-project directory.
- Project Structure:
- Navigate to the project directory and explore its structure:
src/main/java/
: Your Java source files.src/main/resources/
: Configuration files and resources.src/test/java/
: Your test source files.pom.xml
orbuild.gradle
: Project build file.
- Navigate to the project directory and explore its structure:
Add a REST Endpoint
Exploring the project, you should see there is GreetingResource
class that has been automatically generated by the plugin.
If you don’t see it you can manually add it to your project:
package com.codevup.quarkus.demo;
import jakarta.ws.rs.GET;
import jakarta.ws.rs.Path;
import jakarta.ws.rs.Produces;
import jakarta.ws.rs.core.MediaType;
@Path("/hello")
public class GreetingResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
return "Hello Quarkus!";
}
}
Running Your Application
Quarkus offers a development mode that enables hot deployment and testing on the fly.
- Start in Dev Mode:
- In your terminal, navigate to your project root.
- Run
quarkus dev
. This starts your application in development mode. - Your application is now running and accessible at http://localhost:8080.
- Access the REST Endpoint:
- Open a web browser.
- Navigate to http://localhost:8080/hello.
- You should see the text “Hello Quarkus!” displayed.
Conclusion
You’ve successfully created and ran your first Quarkus application with a simple REST endpoint. This demonstrates how Quarkus simplifies the development of microservices and cloud-native applications. In our next article), we will dive deeper into RESTful APIs and we’ll start using Dependency Injection.