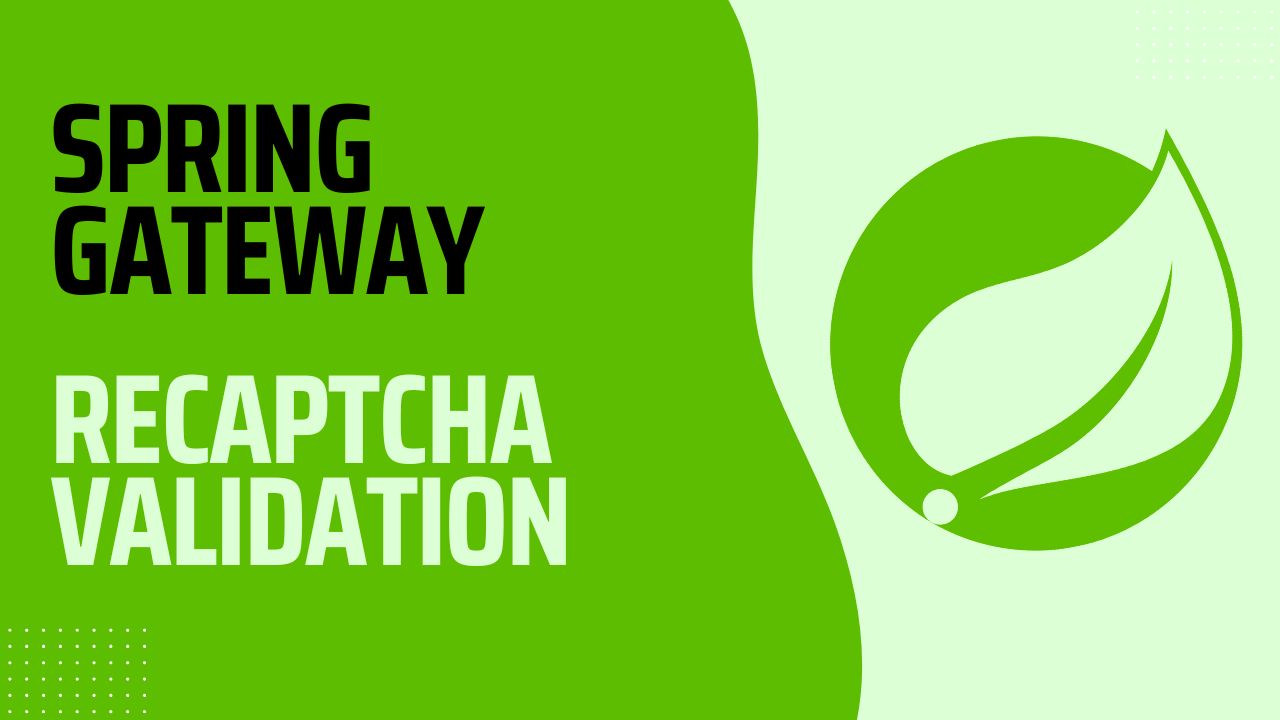
How to add a reCAPTCHA Filter to Spring Gateway
In this tutorial you’ll learn how to add a custom filter to Spring Cloud Gateway with reactive programming, which will filter requests by using reCAPTCHA validation.
Prerequisites
- Setup a small project with spring-cloud-gateway maven dependency
- Create reCAPTCHA keys from Google Admin Console. Guide here
1. Add reCAPTCHA key to properties
You need to save the reCAPTCHA url and secret in the application.yml
google.recaptcha:
url: https://www.google.com/recaptcha/api/siteverify
secret: your-secret-here
2. Extend AbstractGatewayFilterFactory
Let’s define our component by extending AbstractgatewayFilterFactory
, and add google recaptcha parameters and WebClient
to the constructor to be able to do the validation
@Component
public class RecaptchaValidationGatewayFilterFactory
extends AbstractGatewayFilterFactory<RecaptchaValidationGatewayFilterFactory.Config> {
private final WebClient webClient;
private final List<HttpMessageReader<?>> messageReaders;
private final String recaptchaUrl;
private final String recaptchaSecret;
public RecaptchaValidationGatewayFilterFactory(@Value("${google.recaptcha.url}") String recaptchaUrl,
@Value("${google.recaptcha.secret}") String recaptchaSecret) {
super(Config.class);
this.recaptchaUrl = recaptchaUrl;
this.recaptchaSecret = recaptchaSecret;
this.webClient = WebClient.create();
this.messageReaders = HandlerStrategies.withDefaults().messageReaders();
}
@Override
public GatewayFilter apply(Config config) {
return new RecaptchaValidationGatewayFilter(config);
}
public class RecaptchaValidationGatewayFilter implements GatewayFilter, Ordered {
private final Config config;
public RecaptchaValidationGatewayFilter(Config config) {
this.config = config;
}
}
public static class Config {
}
}
3. Call Google url to validate the recaptcha token
Now we need to write a method to call the google url and validate the token. We need to add secret
and response
(recaptcha token) in a request body as it is indicated in google documentation
private Mono<Boolean> validateRecaptchaToken(String recaptchaToken) {
if (recaptchaToken == null) {
return Mono.just(false);
}
var requestBody = new LinkedMultiValueMap<String, String>();
requestBody.add("secret", recaptchaSecret);
requestBody.add("response", recaptchaToken);
return webClient.post().uri(recaptchaUrl).bodyValue(requestBody)
.exchangeToMono(response -> response.bodyToMono(RecaptchaResponse.class))
.flatMap(res -> Mono.just(res.isSuccess()))
.onErrorResume(err -> Mono.just(false));
}
4. Write filter logic to handle validation
Let’s call this validateRecaptchaTooken
method with the token taken from the request. In this tutorial I’m assuming that the token is sent in a request body as a recaptcha_token
, but this can be modified to take the token from request parameters or other ways according to your needs.
We also need to handle the response and make the request fail if the validation wasn’t successful
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
return ServerWebExchangeUtils.cacheRequestBody(exchange, (serverHttpRequest) -> {
ServerRequest serverRequest = ServerRequest.create(exchange.mutate().request(serverHttpRequest).build(), messageReaders);
return serverRequest.bodyToMono(Map.class)
.switchIfEmpty(Mono.just(Map.of()))
.flatMap((body) -> validateRecaptchaToken((String) body.get("recaptcha_token"))
.flatMap(isValid -> {
if (isValid) {
return chain.filter(exchange.mutate().request(serverHttpRequest).build());
} else {
return captchaErrorResponse(exchange);
}
}));
});
}
5. Define filter ordering
We also need to add ordering. Ordering depends on your need, but the best way to set it up is to reference the order of other Spring filters. In this case, we’ll run the filter just before redirecting the request, so that the final route won’t be reached if the validation fails
@Override
public int getOrder() {
return RouteToRequestUrlFilter.ROUTE_TO_URL_FILTER_ORDER - 1;
}
6. Register the filter
Now we need to apply this filter to one or multiple routes. In the following steps we’ll se how to do it for application.yml routes or for programmatic routes
Add the filter to property based routes
spring.cloud.gateway.routes:
- id: my-route
uri: https://my-redirected-url.com/
filters:
- RecaptchaValidation
Add the filter programmatically
@Bean
public RouteLocator routes(
RouteLocatorBuilder builder,
LoggingGatewayFilterFactory loggingFactory) {
return builder.routes()
.route("my-route", r -> r.path("/**")
.filters(f ->
ffilter(recaptchaValidationGatewayFilterFactory.apply(new RecaptchaValidationGatewayFilterFactory.Config())))
.uri("https://my-redirected-url.com/"))
.build();
}
Conclusion
We learned how to apply a filter a request by validating a reCAPTCHA token before routing to the defined url, and modify the response based on the validation result. Here is the full filter code:
@Component
public class RecaptchaValidationGatewayFilterFactory
extends AbstractGatewayFilterFactory<RecaptchaValidationGatewayFilterFactory.Config> {
private final WebClient webClient;
private final List<HttpMessageReader<?>> messageReaders;
private final String recaptchaUrl;
private final String recaptchaSecret;
public RecaptchaValidationGatewayFilterFactory(@Value("${google.recaptcha.url}") String recaptchaUrl,
@Value("${google.recaptcha.secret}") String recaptchaSecret) {
super(Config.class);
this.recaptchaUrl = recaptchaUrl;
this.recaptchaSecret = recaptchaSecret;
this.webClient = WebClient.create();
this.messageReaders = HandlerStrategies.withDefaults().messageReaders();
}
@Override
public GatewayFilter apply(Config config) {
return new RecaptchaValidationGatewayFilter(config);
}
public class RecaptchaValidationGatewayFilter implements GatewayFilter, Ordered {
private final Config config;
public RecaptchaValidationGatewayFilter(Config config) {
this.config = config;
}
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
return ServerWebExchangeUtils.cacheRequestBody(exchange, (serverHttpRequest) -> {
ServerRequest serverRequest = ServerRequest.create(exchange.mutate().request(serverHttpRequest).build(), messageReaders);
return serverRequest.bodyToMono(Map.class)
.switchIfEmpty(Mono.just(Map.of()))
.flatMap((body) -> validateRecaptchaToken((String) body.get(config.getParamName()))
.flatMap(isValid -> {
if (isValid) {
return chain.filter(exchange.mutate().request(serverHttpRequest).build());
} else {
return captchaErrorResponse(exchange);
}
}));
});
}
@Override
public int getOrder() {
return RouteToRequestUrlFilter.ROUTE_TO_URL_FILTER_ORDER - 1;
}
private Mono<Boolean> validateRecaptchaToken(String recaptchaToken) {
if (recaptchaToken == null) {
return Mono.just(false);
}
var requestBody = new LinkedMultiValueMap<String, String>();
requestBody.add("secret", recaptchaSecret);
requestBody.add("response", recaptchaToken);
return webClient.post().uri(recaptchaUrl).bodyValue(requestBody)
.exchangeToMono(response -> response.bodyToMono(RecaptchaResponse.class))
.flatMap(res -> Mono.just(res.isSuccess()))
.onErrorResume(err -> Mono.just(false));
}
private Mono<Void> captchaErrorResponse(ServerWebExchange exchange) {
ServerWebExchangeUtils.setResponseStatus(exchange, HttpStatus.OK);
byte[] bytes = "Your error response here".getBytes(StandardCharsets.UTF_8);
DataBuffer buffer = exchange.getResponse().bufferFactory().wrap(bytes);
exchange.getResponse().getHeaders().setContentType(MediaType.APPLICATION_JSON);
return exchange.getResponse().writeWith(Mono.just(buffer))
.then(Mono.defer(() -> exchange.getResponse().setComplete()));
}
}
public static class Config {
}
}