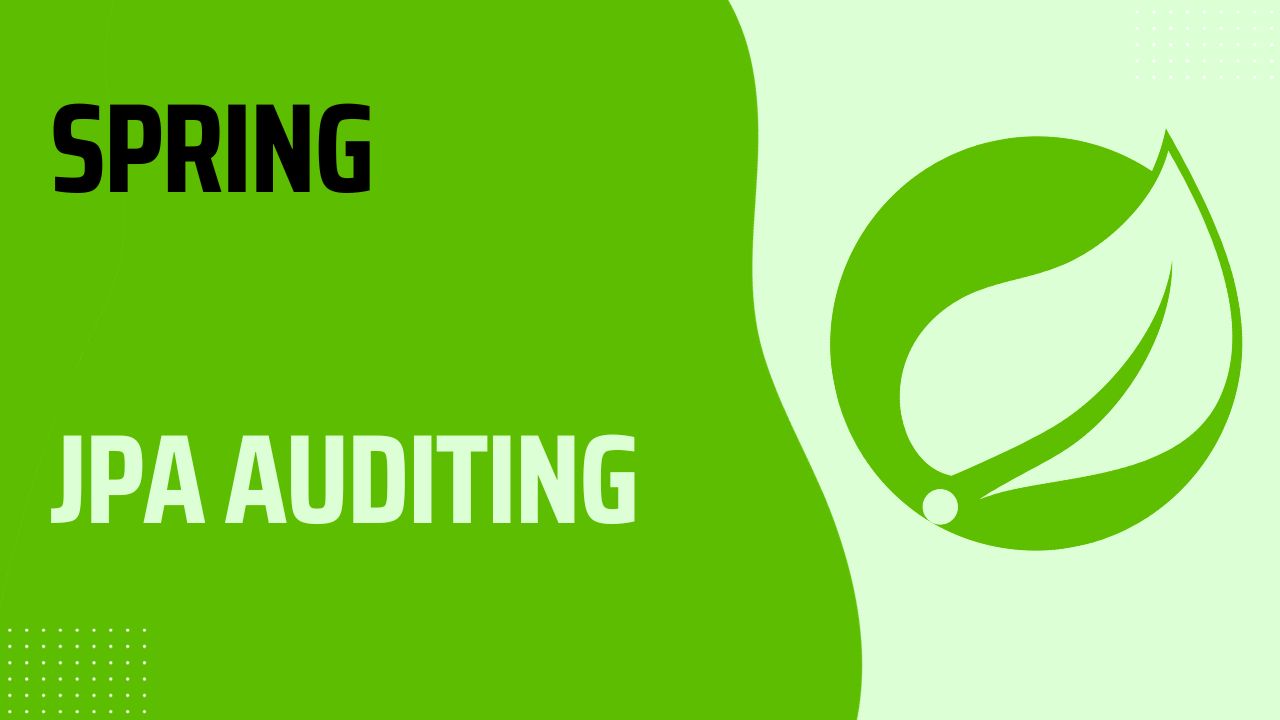
Spring Data JPA Auditing: Tracking Entity Changes
In this tutorial, we’ll look into Spring Data JPA’s auditing capabilities. In particular we’ll show how to keep track of changes made to entities. This feature is especially helpful for applications that require an audit trail or want to monitor the changes to database entities over time.
1. Prerequisites
- Familiarity with Java and Spring Boot.
- Spring Boot project with Spring Data JPA and a configured database.
2. Introduction to JPA Auditing
Spring Data JPA Auditing provides annotations that automatically populate fields like:
@CreatedBy
and@LastModifiedBy
: Track the user who created or last modified the entity.@CreatedDate
and@LastModifiedDate
: Record the timestamp of creation and modification.
3. Setting up JPA Auditing
- Add Dependencies: Ensure you have the
spring-boot-starter-data-jpa
dependency in yourpom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
- Enable JPA Auditing: To activate auditing, annotate your main application or configuration class with
@EnableJpaAuditing
@SpringBootApplication
@EnableJpaAuditing
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
4. Creating Audit Entities
- Base Audit Class: Create a base class that other entities can inherit. This base class will contain audit-related fields
import org.springframework.data.annotation.CreatedBy;
import org.springframework.data.annotation.CreatedDate;
import org.springframework.data.annotation.LastModifiedBy;
import org.springframework.data.annotation.LastModifiedDate;
import org.springframework.data.jpa.domain.support.AuditingEntityListener;
import javax.persistence.EntityListeners;
import javax.persistence.MappedSuperclass;
import java.time.LocalDateTime;
@MappedSuperclass
@EntityListeners(AuditingEntityListener.class)
public abstract class Auditable<U> {
@CreatedBy
protected U createdBy;
@CreatedDate
protected LocalDateTime createdDate;
@LastModifiedBy
protected U lastModifiedBy;
@LastModifiedDate
protected LocalDateTime lastModifiedDate;
// Getters and setters...
}
- Using the Audit Class: To make an entity auditable, extend the base audit class
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Book extends Auditable<String> {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
// Getters, setters, and other fields...
}
5. Displaying Audited Data
With the audit fields populated automatically, you can easily retrieve and display this information in your services or controllers. For instance:
@RestController
@RequestMapping("/books")
public class BookController {
@Autowired
private BookRepository bookRepository;
@GetMapping("/{id}/audit")
public ResponseEntity<?> getAuditData(@PathVariable Long id) {
return bookRepository.findById(id)
.map(book -> ResponseEntity.ok(Map.of(
"createdBy", book.getCreatedBy(),
"createdDate", book.getCreatedDate(),
"lastModifiedBy", book.getLastModifiedBy(),
"lastModifiedDate", book.getLastModifiedDate())))
.orElse(ResponseEntity.notFound().build());
}
}
6. Conclusion
Spring Data JPA Auditing simplifies the process of tracking entity changes in your application. By leveraging a few annotations and a base audit class, entities can automatically capture essential metadata about their creation and modification. This tutorial provided a basic introduction, but there’s much more to explore, including integrating with Spring Security for more comprehensive user tracking. Happy coding!